- Published on
Solidity - Function and Varible visibility
- Authors
- Name
- Saikrishna Reddy
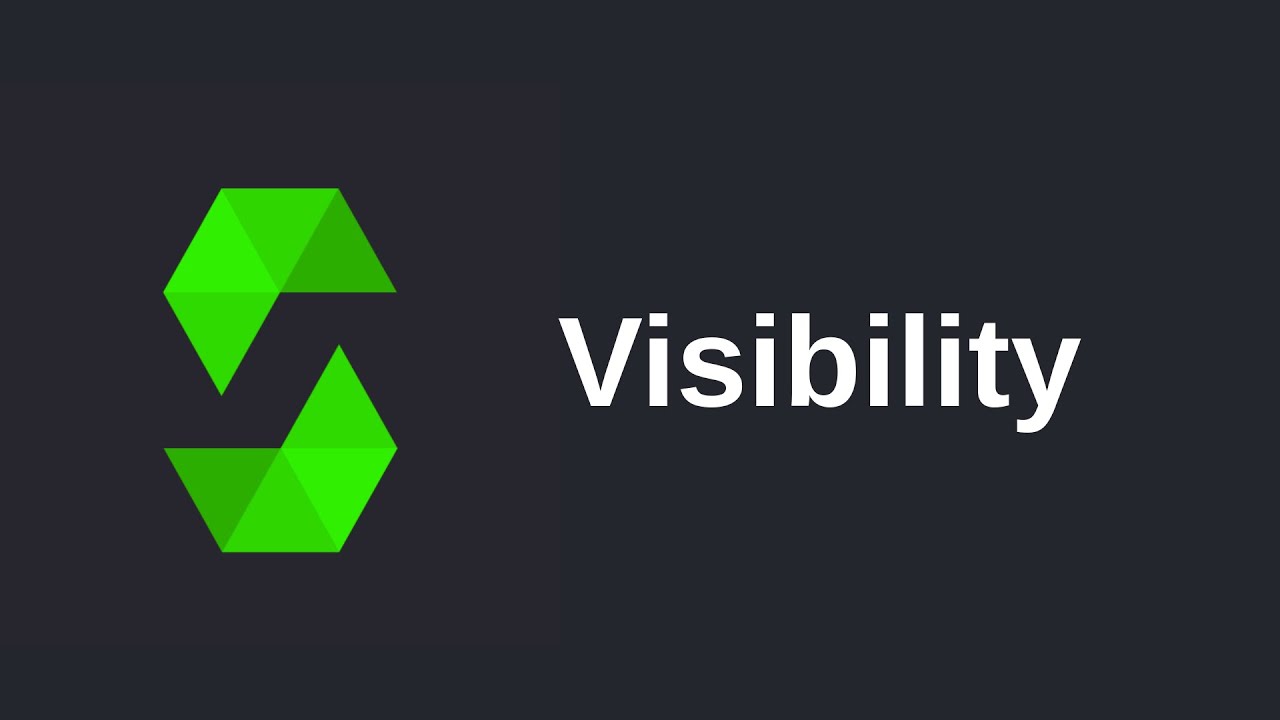
If a developer doesn’t use the right function visibility type, or if no visibility model is specified in their code, the contract's default public visibility is exposed to potentially exploitable security vulnerabilities. Apart from the security implications of not having function visibility specified, smart contracts might not work as intended since the functions will be working without proper instructions.
private :
- This function can only be called from inside the contract.
- Cannot be called from third party contract.
- Cannot be called from derived contract.
contract Parent { function privateMethod() private pure returns(string memory) { return "Working!"; } function callPrivateMethod() public pure returns(string memory){ string memory result = privateMethod(); return result; } } contract B is Parent { function callPrivateMethodFromChild() public pure returns(string memory){ string memory result = privateMethod();//this line throws an error return result; } }
internal :
- This function can be called from inside the contract. and
- can also be called from the derived contract.
contract Parent { function internalMethod() internal pure returns(string memory) { return "Working!"; } function callInternalMethod() public pure returns(string memory){ string memory result = internalMethod(); return result; } } contract B is Parent { function callInternalMethodFromChild() public pure returns(string memory){ string memory result = internalMethod(); return result; } }
external :
- This function can be called from outside/third-party contract only.
- cannot be called from derived contract or inside the contract.
contract Parent { function externalMethod() external pure returns(string memory) { return "Working!"; } function callExternalMethod() public pure returns(string memory){ string memory result = externalMethod(); //this line throws an error return result; } } contract B is Parent { function callExternalMethodFromChild() public pure returns(string memory){ string memory result = externalMethod(); //this line throws an error : ////DeclarationError: Undeclared identifier. return result; } }
public :
- This function can be called from outside/third-party, derived contract and from inside/main the contract.
contract Parent { function publicMethod() public pure returns(string memory) { return "Working!"; } function callPublicMethod() public pure returns(string memory){ string memory result = publicMethod(); return result; } } contract B is Parent { function callPublicMethodFromChild() public pure returns(string memory){ string memory result = publicMethod(); return result; } }
If the visibility modifier of a function is not explicitly declared in the code, the function is set to public visibility by default. Leaving the function visibility blank and relying on the Solidity compiler to use the default visibility is not a recommended best practice.
So use : Least previlage principle
- order for using function visibily is : private > internal > external > public. _ first use private, then see if contracts works, if not then use internal. _ if internal dont works, then use external. _ if external also wont work, then use public as the last option. _ this way we can avoid some of the security vulnerabilities. The difference between function visibility modifiers and state variable visibility modifiers is that state variables do not have the external visibility modifier option.
State variable visibility modifiers
State variables are variables whose values are permanently stored in contract storage, which holds data persistently between function calls. Like function visibility modifiers, state variables also have visibility modifiers which are Public, Private and Internal.
State variables are declared in the contract section of the program. The variable data type is specified and after that, the visibility modifier is assigned to the variable.
When the variable visibility is not defined, the default value is internal.
1. Public
A state variable with the public modifier can be accessed by any contract in the application. If a variable is declared as public, the data stored in it can be read by the main contract, a derived contract, or an external contract.
2. Internal
State variables declared with the internal modifier can only be accessed within the contract in which they were defined and by a derived contract. A third party or another external contract cannot access the storage of the data defined as an internal variable.
3. Private
If a state variable is private, only the main contract in which they were declared can call them. The private visibility modifier restricts access from other parties apart from the main contract so that the data it holds is protected.